How to Use wp_redirect in WordPress
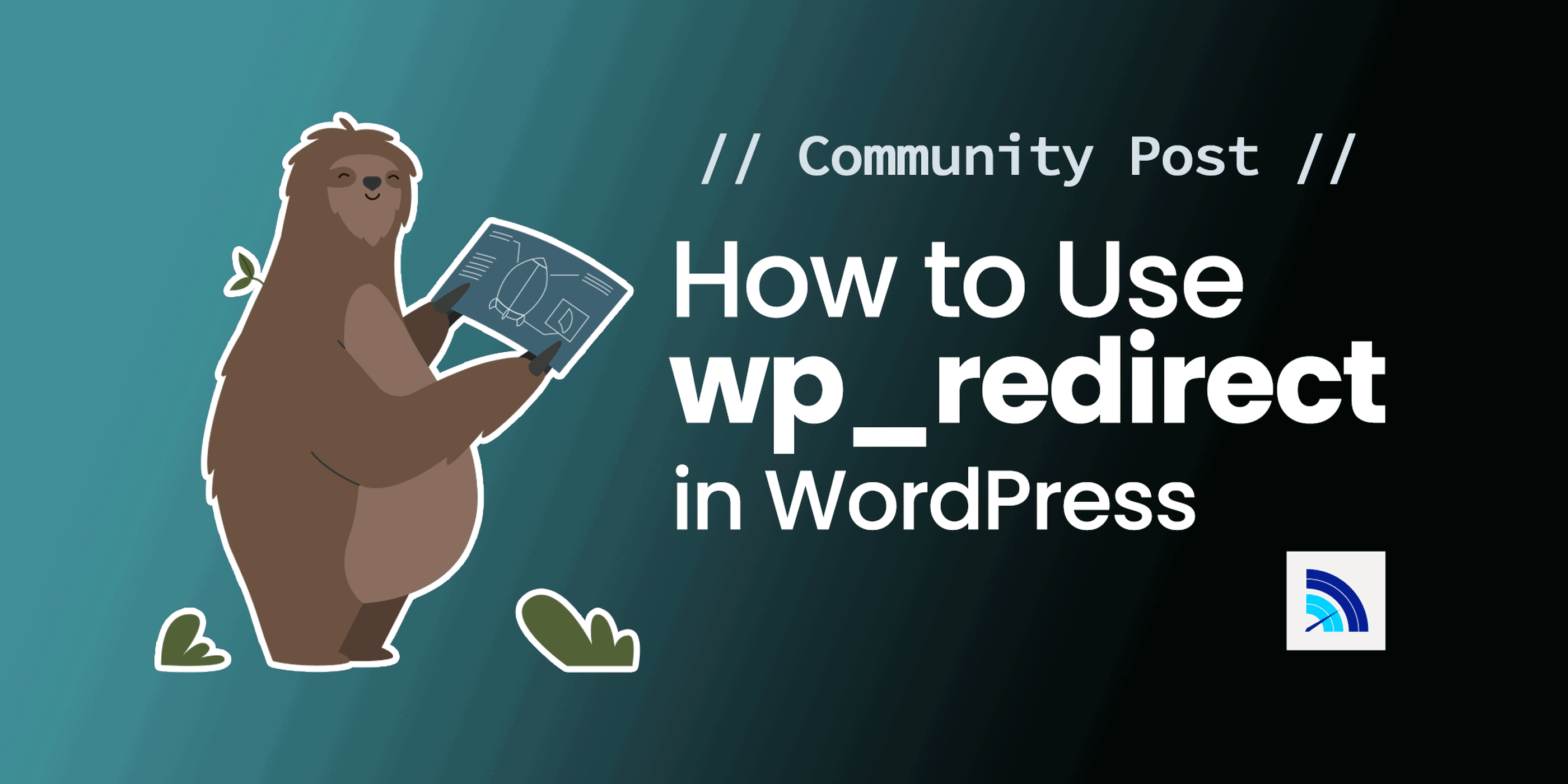
You can use the wp_redirect function to initiate a redirect at different points on your WordPress site. And in this post, I’ll teach you how to use this function and how it works under the hood.
Before we continue, let me explain how the wp_redirect function works.
How the wp_redirect Function Works
You can find the wp_redirect function in the wp-includes/pluggable.php
file with several other helper functions. The job of this function is to redirect your WordPress site to another page. For example, you can use this function to redirect from one page to another based on some condition or your specific need. You may also use it to redirect users to an external website.
Nature of the wp_redirect Function
At the time of this writing, the following code shows how the wp_redirect function is implemented:
function wp_redirect( $location, $status = 302, $x_redirect_by = 'WordPress' ) {
global $is_IIS;
/**
* Filters the redirect location.
*
* @since 2.1.0
*
* @param string $location The path or URL to redirect to.
* @param int $status The HTTP response status code to use.
*/
$location = apply_filters( 'wp_redirect', $location, $status );
/**
* Filters the redirect HTTP response status code to use.
*
* @since 2.3.0
*
* @param int $status The HTTP response status code to use.
* @param string $location The path or URL to redirect to.
*/
$status = apply_filters( 'wp_redirect_status', $status, $location );
if ( ! $location ) {
return false;
}
if ( $status < 300 || 399 < $status ) {
wp_die( __( 'HTTP redirect status code must be a redirection code, 3xx.' ) );
}
$location = wp_sanitize_redirect( $location );
if ( ! $is_IIS && 'cgi-fcgi' !== PHP_SAPI ) {
status_header( $status ); // This causes problems on IIS and some FastCGI setups.
}
/**
* Filters the X-Redirect-By header.
*
* Allows applications to identify themselves when they're doing a redirect.
*
* @since 5.1.0
*
* @param string $x_redirect_by The application doing the redirect.
* @param int $status Status code to use.
* @param string $location The path to redirect to.
*/
$x_redirect_by = apply_filters( 'x_redirect_by', $x_redirect_by, $status, $location );
if ( is_string( $x_redirect_by ) ) {
header( "X-Redirect-By: $x_redirect_by" );
}
header( "Location: $location", true, $status );
return true;
}
Now, let’s walk through how the function works.
Parameters: wp_redirect( $location, $status = 302, $x_redirect_by = ‘WordPress’ )
In the code above, the wp_redirect function accepts three parameters that include location, status, and x_redirect_by. Both status and x_redirect_by are optional. That means you can still use the function by specifying only the URL you wish to redirect to. In a case like that, a redirect with the 302 status code will be initiated. There are different redirect codes with different purposes and use cases, and the 302 status code means a page has been temporarily moved. A status code of 301 means the page or resource has been moved permanently.
Procedures
The rest of the function verifies the parameters provided by the user to determine if the redirect should be performed or canceled or determine what type of redirect to do.
The function will return false
when the redirect is canceled. Otherwise, it will return true
.
How to Use wp_redirect to Redirect to Another Page
Now that you know about the wp_redirect function and its internal workings, I’ll walk you through a step-by-step guide on how to redirect to another page using the function.
Step 1: Create a Function to Call wp_redirect
First, create a simple function that calls the wp_redirect. To do that, open your current theme’s functions.php and add the following code at the end of the file:
function redirect_to_thank_you() {
wp_redirect('thank-you');
exit();
}
For this example, you’re modifying the default twentytwentytwo theme. Hence the location for functions.php is wp-content/themes/twentytwentytwo.
Step 2: Attach the Function to a Hook
For this tutorial, you’ll be performing a redirect using an action hook. Action hooks allow you to run functions once a specific event occurs in WordPress. For example, you can run a function every time a new post is submitted. However, in this example, you’ll redirect the user to a custom thank you page after they successfully submit a comment.
In order to determine when a user submits a new comment, you’ll use the comment_post action. So, attach the function you created in step 1 to that action using the add_action()
function. To do that, still within the theme’s functions.php file, add the following code to the end of the file:
add_action('comment_post', 'redirect_to_thank_you');
Step 3: Create a Thank You Page
Before we continue, add a new thank you page to your WordPress site.
To do that, open the WordPress admin dashboard (for example yoursite.com/wp-admin) and navigate to Pages > Add New.
Enter “Thank You” as the page title, then add a message to the body. Once you’re done, make sure the URL slug under Permalink is “thank-you”, then hit Publish to finish.
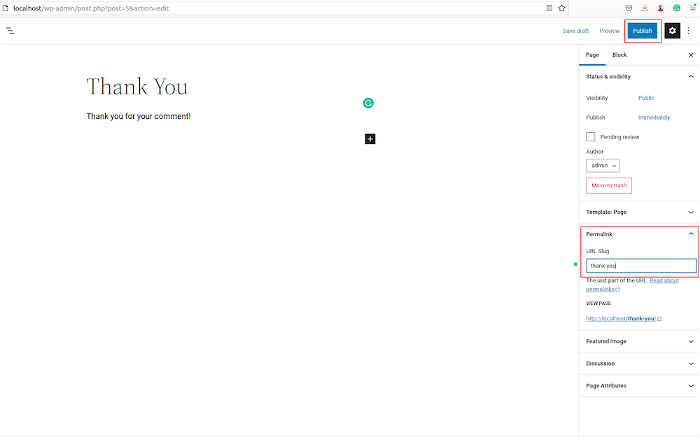
Step 4: Post a Comment
In order to test the work you’ve done so far, try to post a new comment.
Go to any post on your blog, type some comments in the text field, and submit. You should be redirected to a /thank-you page within your site. If this page does not exist, you’ll see a standard WordPress 404 error page.
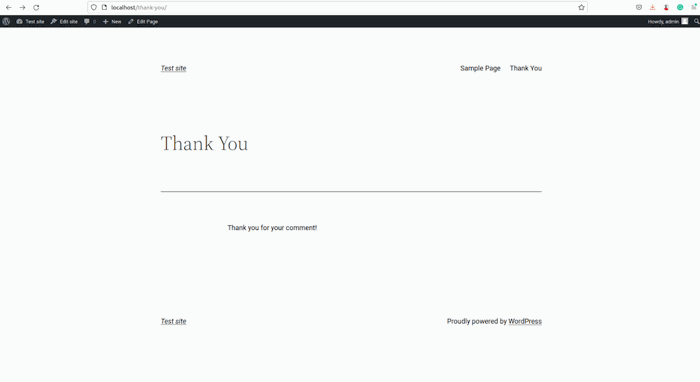
Step 5: Redirect to an External Page
Besides redirecting users to another page on your website, you can also use the wp_redirect function to redirect users to another website. For example, you can redirect all the traffic on your site to another site using a hook that fires the moment a page is requested. You can do a redirect that takes users to google.com just to test how this works.
First, create another function that calls wp_redirect. This is similar to what you did in step 1 above. The following code shows the function:
function redirect_to_google() {
wp_redirect('https://google.com');
exit();
}
To finish, create a new action hook for when you want the redirect to occur and attach the above function. Here’s an example using the after_setup_theme
action:
add_action( 'after_setup_theme', 'redirect_to_google' );
Once you’re done, try loading any page on your website, and you should be redirected to Google.com.
Troubleshooting wp_redirect
You can run into several issues while using wp_redirect. Some of the challenges you may run into due to the wrong use of wp_redirect include performance issues like your site running slower, or even poor impact on your SEO.
Some of these issues include looping redirects and PHP header errors. Let’s take a look at an example of how a misconfigured wp_redirect may lead to a PHP header error.
How Misconfigured Redirect Can Cause Performance Issues
In PHP, you can redirect user requests using header('location: TARGET_URL')
. However, this function requires that your page outputs no data before calling it. In a case when you call the wp_redirect function from a place where WordPress has already outputted some HTML, you may run into an error with the famous “headers already sent …” message. This type of error can cause your redirect to fail completely and can flood your error logs with warning messages for every request sent to the affected URL.
To fix this problem, always attach the wp_redirect function to a hook that’s triggered before any HTML is rendered.
Best Practices
The following are some best practices for using wp_redirect
to redirect pages in WordPress:
- Don’t call the wp_redirect function after any code that prints output to the screen. Doing so may lead to PHP header errors.
- WordPress has several hooks for plugin development and customizing your site, and you should use the correct hook to initiate your redirect.
- Always use the right redirect status code as using the wrong code may break your SEO.
- Avoid chaining redirects or redirect loops as this may lead to a poor user experience and impact your SEO.
- Always call the
exit()
function after callingwp_redirect()
.
Summary
In this post, you saw how the wp_redirect
function works. It’s a utility function that makes it easy to direct users of your WordPress site from one page to another. The post also provided a tutorial on how to redirect a user to another page after an action like posting a new comment. It’s also possible to redirect users to an external site using the wp_redirect function, and we showed you an example of how to do that. Finally, we listed some best practices and how to troubleshoot redirects.
If you’re interested in web performance and making better experiences for your users, check out Request Metrics client-side observability tools.
This post was written by Pius Aboyi. Pius is a mobile and web developer with over 4 years of experience building for the Android platform. He writes code in Java, Kotlin, and PHP. He loves writing about tech and creating how-to tutorials for developers.